CP164 Study Guide - Final Guide: Data Element, Methamphetamine
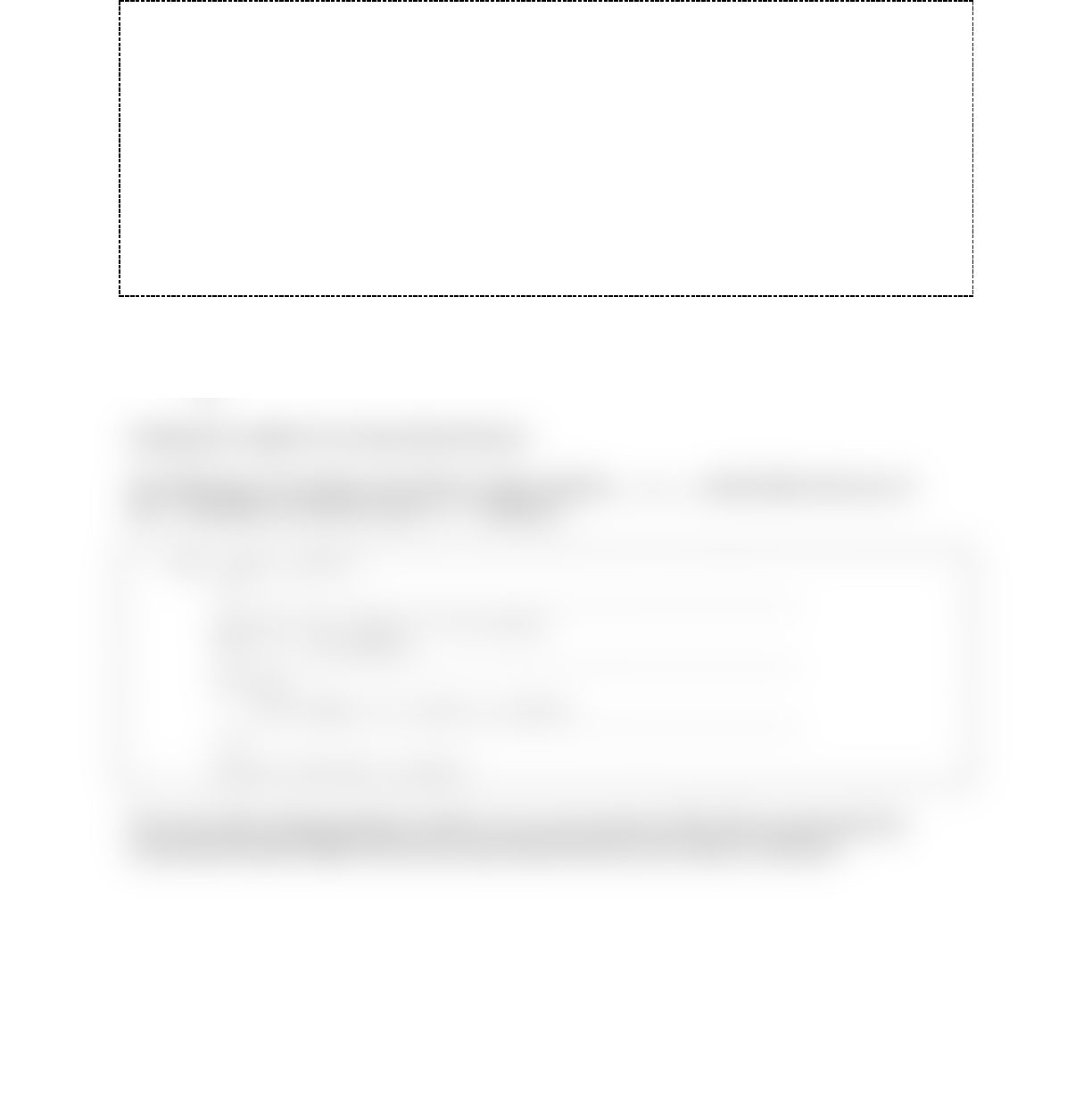
Notes - The Array-Based Queue
Implementation
Inserting Items Into an Array-Based Queue
def insert(self, value):
"""
-------------------------------------------------------
Adds a copy of value to the rear of the queue.
Use: queue.insert(value)
-------------------------------------------------------
Parameters:
value - a data element (?)
Returns:
None
-------------------------------------------------------
"""
self._values.append(deepcopy(value))
return
insert simply uses the Python list method append to add value to the end of
the _values list. Except for the name, this method is identical to the Stack push method
including the use of deepcopy.
Getting the Length of an Array-Based Queue
The following code defines the Python magic method __len__, which allows the use of
the lenfunction, as shown in the Use: comment.
def __len__(self):
"""
-------------------------------------------------------
Returns the length of the queue.
Use: n = len(queue)
-------------------------------------------------------
Returns:
the number of values in queue.
-------------------------------------------------------
"""
return len(self._values)
The rest of the implementation is left to you as an exercise. Note that in particular the
array-based Queue differs from the array-based Stack in how data is removed.
find more resources at oneclass.com
find more resources at oneclass.com
Document Summary
Inserting items into an array-based queue def insert(self, value): Adds a copy of value to the rear of the queue. None self. _values. append(deepcopy(value)) return insert simply uses the python list method append to add value to the end of the _values list. Except for the name, this method is identical to the stack push method including the use of deepcopy. The following code defines the python magic method __len__, which allows the use of the lenfunction, as shown in the use: comment. def __len__(self): Returns: the number of values in queue. return len(self. _values) The rest of the implementation is left to you as an exercise. Note that in particular the array-based queue differs from the array-based stack in how data is removed. The array-based implementation of the queue that we have already seen is very simple to implement, but that simplicity - and the use of the python list - hides a very inefficient implementation.