Engineering Science 1036A/B Lecture Notes - Lecture 12: Newline, Semicolon, Function Prototype
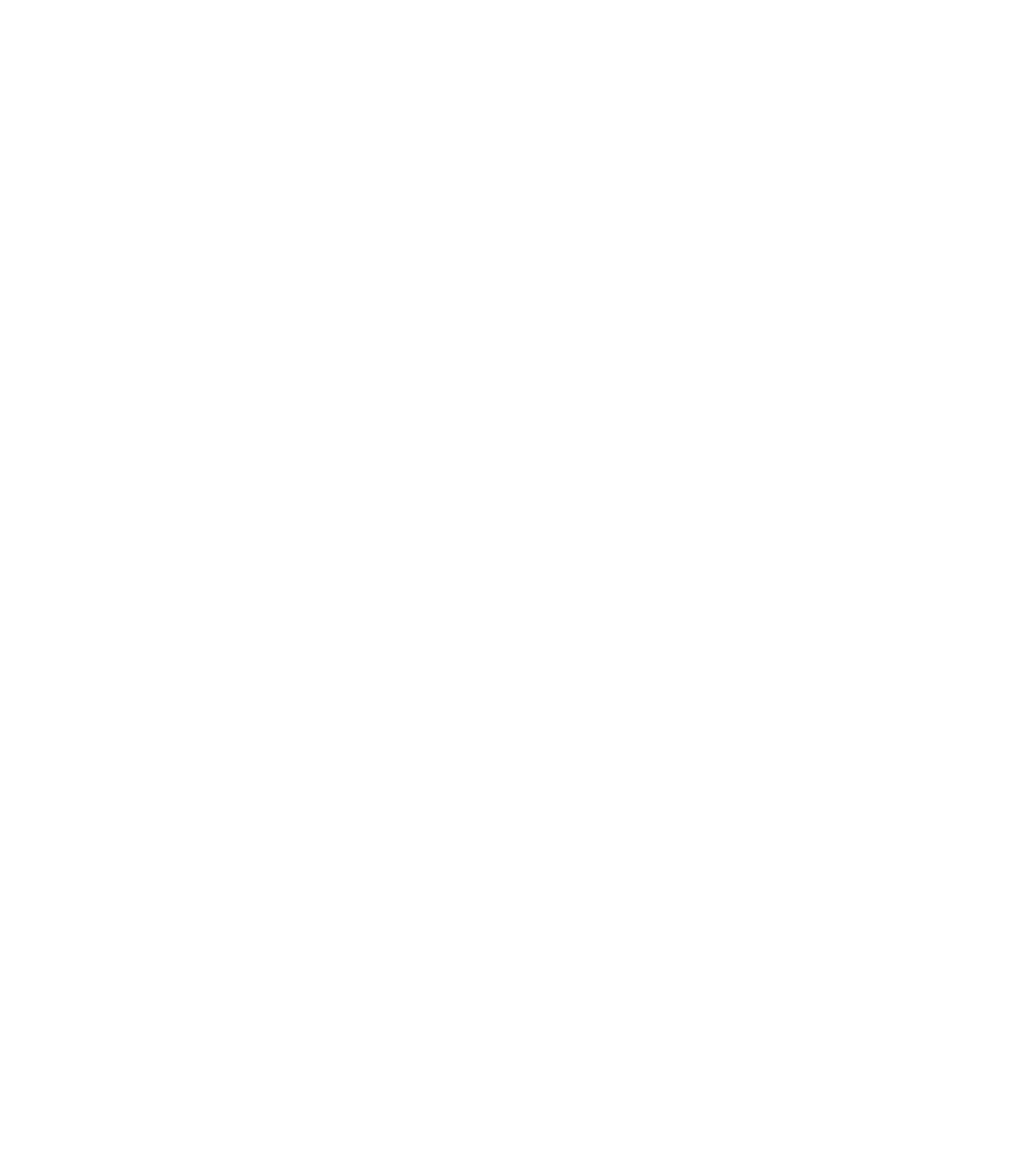
Function: collection of statements that are grouped together to perform an
operation
•
Modular programming approach: Complex programs can be broken down into
sub tasks, each of which is easy to manage and implement
Multiple programmers can be involved
○
Testing/debugging/maintenance becomes easy
○
Code duplication is reduced
○
Information hiding: hide the implementation from the user
○
•
2 function types:
Pre-defined
○
Programmer/user defined
○
•
Terminology of Functions
Function prototype
Function declaration with its signature/header
○
•
Function call
•
Function arguments
Used in function call
○
•
Function definition
Function header
○
Function body
○
•
Formal parameters
Used in function definition
○
•
Function header/signature
Return type
○
Function name
○
Parameter list
Data type
§
Order
§
Number
§
○
•
User-Defined Functions
Programmer writes the entire function definition
(name, return type, number and type of parameters and body)
○
•
Defined outside the main() function either above or below
Function max has been defined above main() as a separate entity,
and has been called from the main()
§
○
Function max has been defined underneath main() ,and has been
called from the main()
§
Function prototype/declaration is required above the main()
§
○
•
A function signature has a return type (data-type or void), a name and a formal
parameter list
int gradePAverage(string name, int numberOfSubjects);
○
void myFunction(int x);
○
void doNothing();
○
int main(void)
○
Return type
A function may or may not return a value back to the calling
function
§
If a function returns a value, the return-type would be the data type
of that value
§
If the function does not return a value, the return type is the
keyword void
§
○
Function name
Cannot be a keyword and it has to be a valid C++ identifier
§
○
Formal parameter list
The variables defined after the function name enclosed by round
bracket are known as formal parameter
§
Comma separated list of variable declarations (data type followed
by name)
§
If no parameters needs to be passed in to the function, the list can
be left empty or the keyword void can be used there
§
○
•
Function definition contains function header and function body•
When a function is called/invoked, no value (such as void) or different types of
values can be passed (pass by value) to a function
These values which are passed to a function are referred as actual
parameters or arguments
Arguments must agree with the formal parameters in order,
number and data type, but identifiers (variable names) in the
function header and in the argument list can be different
§
○
•
Function Prototype
A function declaration•
Contains:
Return-type, function name, and a parameter list, followed by a
semicolon (because it is a declaration)
int gradePAverage(string name, int
numberOfSubjects);
Can also be written without using names of the parameters:
int gradePAverage(string, int);
®
□
§
○
•
Declaration/prototype is made outside and above the main function
Exception: if the function definition is placed above the main() function
definition, function declaration becomes optional
○
•
Calling (Invoking) Functions
"call" or "invoke" a function when you want to use it•
Flow of control is transferred to its body•
Values of parameters are passed along•
Formal parameters must agree with arguments in order, number, and data type,
but parameter names can be different
•
If you call a function from cout-statement, the function call will have the higher
precedence than the cout statement
•
At the end of body, flow of control is transferred back•
A function can return a single object to the calling program
The function header declares the type of object to be returned
○
A return statement is required in the body of the function
○
•
Parameter Passing
Pass by value
Default behavior
○
Formal parameter receives a copy of the argument
○
Changes to the formal parameter content do not affect the original object
value that passed via argument
○
•
Pass by reference
Changing formal parameter content will change the original object value
that was passed via argument
○
•
Void Functions
A function that does not return a value to the calling program is known as void
function
•
Void function examples:
void myFunction(int a, double b);
○
void myFunction();
○
•
In the function definition of a void function, return statement is optional•
If a return statement is used in the void function, it has the following form
return;
○
•
Pre-defined Functions
Stored in standard libraries
<cmath>
○
•
Already defined•
User only needs to know how to use/call it•
Pre-defined function example
Both sqrt and pow function are predefined functions which have been
defined in the <cmath> library
○
List of Pre-defined Functions
Pre-defined Function: int rand(void)
rand function is a standard library function, available in C-standard library
<stdlib.h>
Don’t need to add the <stdlib.h> library
○
•
Returns a pseudo-random number between 0 and RAND_MAX•
RAND_MAX is a symbolic constant defined in the standard library
Has a value of at least 32767
○
•
Generate a random number using rand()
The statement int x= rand(); assigns a random number between 0 and
RAND_MAX to x
•
To generate a random number between 0 and an integer is to use the modulus
operator
To generate a random number between 0 and 5 inclusive, use rand()%6
○
•
If random numbers starting at a value other than zero are to be generated, the
formula is modified by adding the starting value
The roll of a die will yield a 1,2,3,4,5, or 6, to simulate the roll of a die, use
1 + rand() % 6
○
•
In general, to generate numbers from min to max inclusive, use min + rand()
% (max –min + 1)
•
•
Pre-defined Function: void srand (unsigned int x)
Used to get different random numbers each time the program is run•
The srand function is said to “seed” the function rand•
It takes an unsigned integer argument
A different sequence of numbers can be generated by using different seed
values
○
•
•
Predefined function: time_t time(time_t* y)
Incude <ctime> library•
Used to enter seed values automatically•
The time function returns the time of day in seconds•
number of seconds since 1 January 1970•
The return value changes in each second•
Using “NULL” as the parameter causes the time function to return the value of
the seed
•
•
Predefined function: getline()
Syntax:
void getline(cin, string_variable_name);
○
•
The statement cin.ignore(); will always be required before using the
getline function if any cin operation is carried out earlier in the same
programming block
cin.ignore(); statement skips the newline (‘\n’) character in the
input buffer ()
○
•
•
C++ Functions
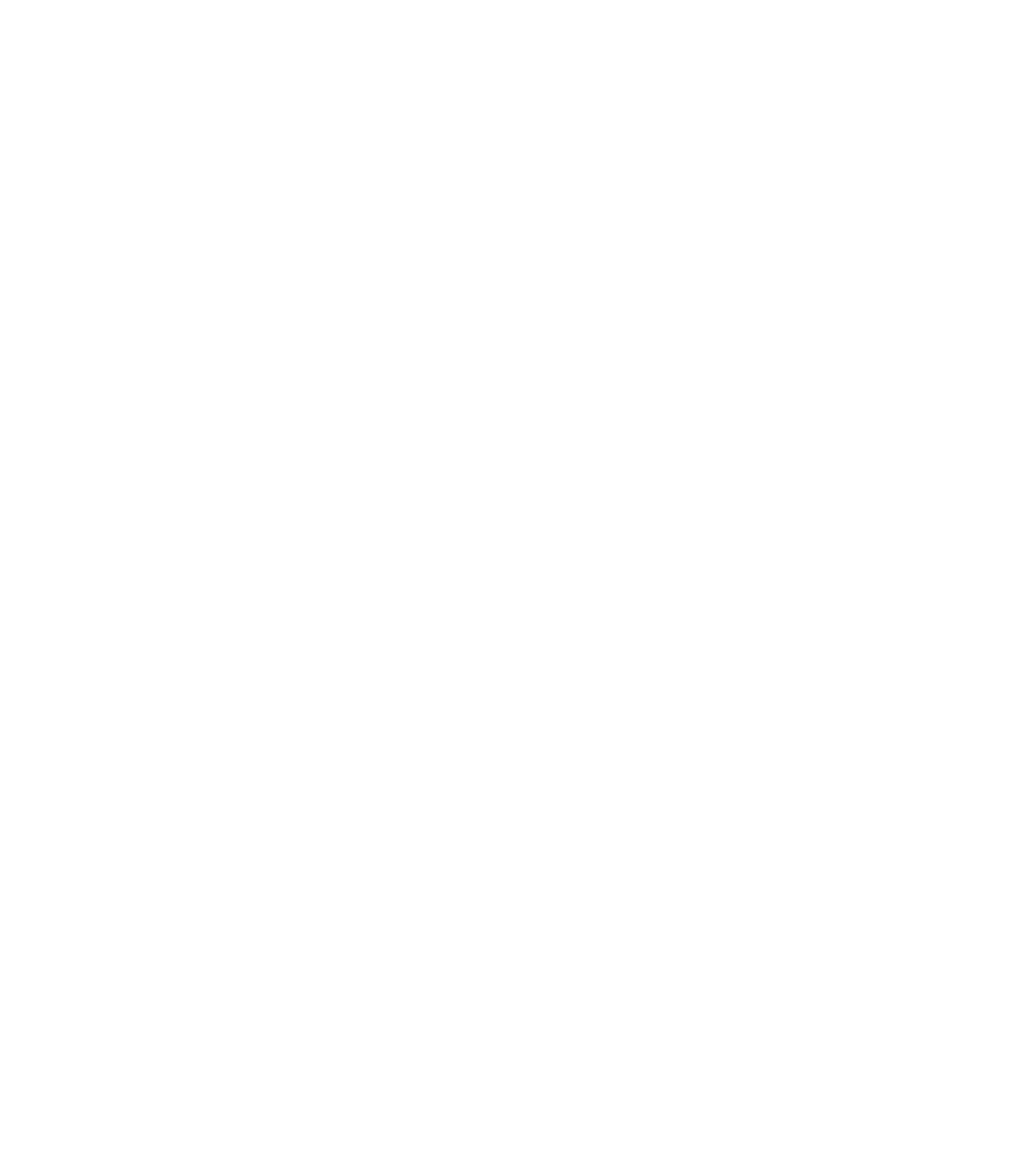
Function: collection of statements that are grouped together to perform an
operation
•
Modular programming approach: Complex programs can be broken down into
sub tasks, each of which is easy to manage and implement
Multiple programmers can be involved
○
Testing/debugging/maintenance becomes easy
○
Code duplication is reduced
○
Information hiding: hide the implementation from the user
○
•
2 function types:
Pre-defined
○
Programmer/user defined
○
•
Terminology of Functions
Function prototype
Function declaration with its signature/header
○
•
Function call•
Function arguments
Used in function call
○
•
Function definition
Function header
○
Function body
○
•
Formal parameters
Used in function definition
○
•
Function header/signature
Return type
○
Function name
○
Parameter list
Data type
§
Order
§
Number
§
○
•
User-Defined Functions
Programmer writes the entire function definition
(name, return type, number and type of parameters and body)
○
•
Defined outside the main() function either above or below
Function max has been defined above main() as a separate entity,
and has been called from the main()
§
○
Function max has been defined underneath main() ,and has been
called from the main()
§
Function prototype/declaration is required above the main()
§
○
•
A function signature has a return type (data-type or void), a name and a formal
parameter list
int gradePAverage(string name, int numberOfSubjects);
○
void myFunction(int x);
○
void doNothing();
○
int main(void)
○
Return type
A function may or may not return a value back to the calling
function
§
If a function returns a value, the return-type would be the data type
of that value
§
If the function does not return a value, the return type is the
keyword void
§
○
Function name
Cannot be a keyword and it has to be a valid C++ identifier
§
○
Formal parameter list
The variables defined after the function name enclosed by round
bracket are known as formal parameter
§
Comma separated list of variable declarations (data type followed
by name)
§
If no parameters needs to be passed in to the function, the list can
be left empty or the keyword void can be used there
§
○
•
Function definition contains function header and function body•
When a function is called/invoked, no value (such as void) or different types of
values can be passed (pass by value) to a function
These values which are passed to a function are referred as actual
parameters or arguments
Arguments must agree with the formal parameters in order,
number and data type, but identifiers (variable names) in the
function header and in the argument list can be different
§
○
•
Function Prototype
A function declaration•
Contains:
Return-type, function name, and a parameter list, followed by a
semicolon (because it is a declaration)
int gradePAverage(string name, int
numberOfSubjects);
Can also be written without using names of the parameters:
int gradePAverage(string, int);
®
□
§
○
•
Declaration/prototype is made outside and above the main function
Exception: if the function definition is placed above the main() function
definition, function declaration becomes optional
○
•
Calling (Invoking) Functions
"call" or "invoke" a function when you want to use it•
Flow of control is transferred to its body•
Values of parameters are passed along•
Formal parameters must agree with arguments in order, number, and data type,
but parameter names can be different
•
If you call a function from cout-statement, the function call will have the higher
precedence than the cout statement
•
At the end of body, flow of control is transferred back•
A function can return a single object to the calling program
The function header declares the type of object to be returned
○
A return statement is required in the body of the function
○
•
Parameter Passing
Pass by value
Default behavior
○
Formal parameter receives a copy of the argument
○
Changes to the formal parameter content do not affect the original object
value that passed via argument
○
•
Pass by reference
Changing formal parameter content will change the original object value
that was passed via argument
○
•
Void Functions
A function that does not return a value to the calling program is known as void
function
•
Void function examples:
void myFunction(int a, double b);
○
void myFunction();
○
•
In the function definition of a void function, return statement is optional•
If a return statement is used in the void function, it has the following form
return;
○
•
Pre-defined Functions
Stored in standard libraries
<cmath>
○
•
Already defined•
User only needs to know how to use/call it•
Pre-defined function example
Both sqrt and pow function are predefined functions which have been
defined in the <cmath> library
○
List of Pre-defined Functions
Pre-defined Function: int rand(void)
rand function is a standard library function, available in C-standard library
<stdlib.h>
Don’t need to add the <stdlib.h> library
○
•
Returns a pseudo-random number between 0 and RAND_MAX•
RAND_MAX is a symbolic constant defined in the standard library
Has a value of at least 32767
○
•
Generate a random number using rand()
The statement int x= rand(); assigns a random number between 0 and
RAND_MAX to x
•
To generate a random number between 0 and an integer is to use the modulus
operator
To generate a random number between 0 and 5 inclusive, use rand()%6
○
•
If random numbers starting at a value other than zero are to be generated, the
formula is modified by adding the starting value
The roll of a die will yield a 1,2,3,4,5, or 6, to simulate the roll of a die, use
1 + rand() % 6
○
•
In general, to generate numbers from min to max inclusive, use min + rand()
% (max –min + 1)
•
•
Pre-defined Function: void srand (unsigned int x)
Used to get different random numbers each time the program is run•
The srand function is said to “seed” the function rand•
It takes an unsigned integer argument
A different sequence of numbers can be generated by using different seed
values
○
•
•
Predefined function: time_t time(time_t* y)
Incude <ctime> library•
Used to enter seed values automatically•
The time function returns the time of day in seconds•
number of seconds since 1 January 1970•
The return value changes in each second•
Using “NULL” as the parameter causes the time function to return the value of
the seed
•
•
Predefined function: getline()
Syntax:
void getline(cin, string_variable_name);
○
•
The statement cin.ignore(); will always be required before using the
getline function if any cin operation is carried out earlier in the same
programming block
cin.ignore(); statement skips the newline (‘\n’) character in the
input buffer ()
○
•
•
C++ Functions
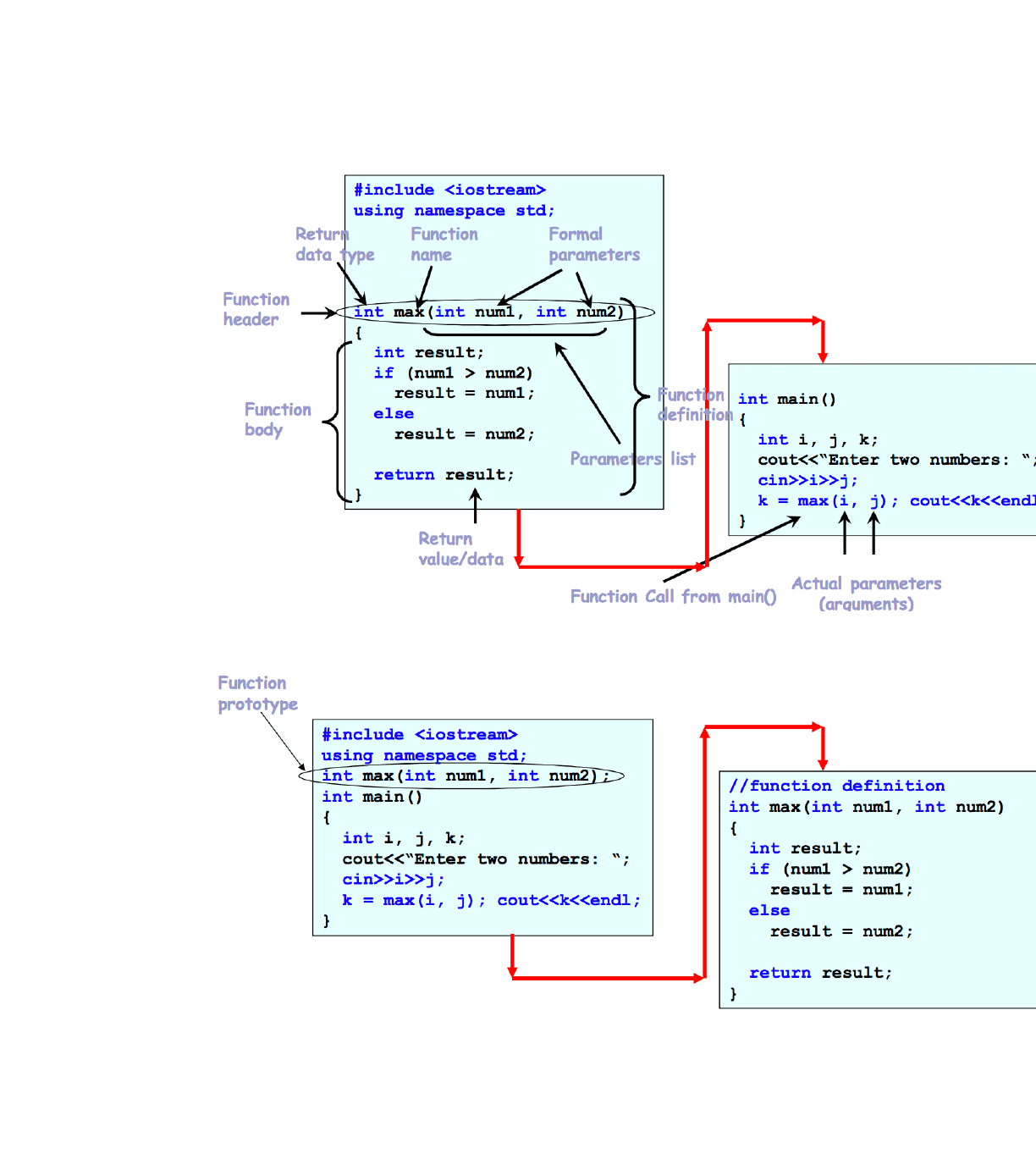
Function: collection of statements that are grouped together to perform an
operation
•
Modular programming approach: Complex programs can be broken down into
sub tasks, each of which is easy to manage and implement
Multiple programmers can be involved
○
Testing/debugging/maintenance becomes easy
○
Code duplication is reduced
○
Information hiding: hide the implementation from the user
○
•
2 function types:
Pre-defined
○
Programmer/user defined
○
•
Terminology of Functions
Function prototype
Function declaration with its signature/header
○
•
Function call•
Function arguments
Used in function call
○
•
Function definition
Function header
○
Function body
○
•
Formal parameters
Used in function definition
○
•
Function header/signature
Return type
○
Function name
○
Parameter list
Data type
§
Order
§
Number
§
○
•
User-Defined Functions
Programmer writes the entire function definition
(name, return type, number and type of parameters and body)
○
•
Defined outside the main() function either above or below
Function max has been defined above main() as a separate entity,
and has been called from the main()
§
○
Function max has been defined underneath main() ,and has been
called from the main()
§
Function prototype/declaration is required above the main()
§
○
•
A function signature has a return type (data-type or void), a name and a formal
parameter list
int gradePAverage(string name, int numberOfSubjects);
○
void myFunction(int x);
○
void doNothing();
○
int main(void)
○
Return type
A function may or may not return a value back to the calling
function
§
If a function returns a value, the return-type would be the data type
of that value
§
If the function does not return a value, the return type is the
keyword void
§
○
Function name
Cannot be a keyword and it has to be a valid C++ identifier
§
○
Formal parameter list
The variables defined after the function name enclosed by round
bracket are known as formal parameter
§
Comma separated list of variable declarations (data type followed
by name)
§
If no parameters needs to be passed in to the function, the list can
be left empty or the keyword void can be used there
§
○
•
Function definition contains function header and function body•
When a function is called/invoked, no value (such as void) or different types of
values can be passed (pass by value) to a function
These values which are passed to a function are referred as actual
parameters or arguments
Arguments must agree with the formal parameters in order,
number and data type, but identifiers (variable names) in the
function header and in the argument list can be different
§
○
•
Function Prototype
A function declaration•
Contains:
Return-type, function name, and a parameter list, followed by a
semicolon (because it is a declaration)
int gradePAverage(string name, int
numberOfSubjects);
Can also be written without using names of the parameters:
int gradePAverage(string, int);
®
□
§
○
•
Declaration/prototype is made outside and above the main function
Exception: if the function definition is placed above the main() function
definition, function declaration becomes optional
○
•
Calling (Invoking) Functions
"call" or "invoke" a function when you want to use it•
Flow of control is transferred to its body•
Values of parameters are passed along•
Formal parameters must agree with arguments in order, number, and data type,
but parameter names can be different
•
If you call a function from cout-statement, the function call will have the higher
precedence than the cout statement
•
At the end of body, flow of control is transferred back•
A function can return a single object to the calling program
The function header declares the type of object to be returned
○
A return statement is required in the body of the function
○
•
Parameter Passing
Pass by value
Default behavior
○
Formal parameter receives a copy of the argument
○
Changes to the formal parameter content do not affect the original object
value that passed via argument
○
•
Pass by reference
Changing formal parameter content will change the original object value
that was passed via argument
○
•
Void Functions
A function that does not return a value to the calling program is known as void
function
•
Void function examples:
void myFunction(int a, double b);
○
void myFunction();
○
•
In the function definition of a void function, return statement is optional•
If a return statement is used in the void function, it has the following form
return;
○
•
Pre-defined Functions
Stored in standard libraries
<cmath>
○
•
Already defined•
User only needs to know how to use/call it•
Pre-defined function example
Both sqrt and pow function are predefined functions which have been
defined in the <cmath> library
○
List of Pre-defined Functions
Pre-defined Function: int rand(void)
rand function is a standard library function, available in C-standard library
<stdlib.h>
Don’t need to add the <stdlib.h> library
○
•
Returns a pseudo-random number between 0 and RAND_MAX•
RAND_MAX is a symbolic constant defined in the standard library
Has a value of at least 32767
○
•
Generate a random number using rand()
The statement int x= rand(); assigns a random number between 0 and
RAND_MAX to x
•
To generate a random number between 0 and an integer is to use the modulus
operator
To generate a random number between 0 and 5 inclusive, use rand()%6
○
•
If random numbers starting at a value other than zero are to be generated, the
formula is modified by adding the starting value
The roll of a die will yield a 1,2,3,4,5, or 6, to simulate the roll of a die, use
1 + rand() % 6
○
•
In general, to generate numbers from min to max inclusive, use min + rand()
% (max –min + 1)
•
•
Pre-defined Function: void srand (unsigned int x)
Used to get different random numbers each time the program is run•
The srand function is said to “seed” the function rand•
It takes an unsigned integer argument
A different sequence of numbers can be generated by using different seed
values
○
•
•
Predefined function: time_t time(time_t* y)
Incude <ctime> library•
Used to enter seed values automatically•
The time function returns the time of day in seconds•
number of seconds since 1 January 1970•
The return value changes in each second•
Using “NULL” as the parameter causes the time function to return the value of
the seed
•
•
Predefined function: getline()
Syntax:
void getline(cin, string_variable_name);
○
•
The statement cin.ignore(); will always be required before using the
getline function if any cin operation is carried out earlier in the same
programming block
cin.ignore(); statement skips the newline (‘\n’) character in the
input buffer ()
○
•
•
C++ Functions
Document Summary
Function: collection of statements that are grouped together to perform an operation. Modular programming approach: complex programs can be broken down into sub tasks, each of which is easy to manage and implement. Information hiding: hide the implementation from the user. Programmer writes the entire function definition (name, return type, number and type of parameters and body) Defined outside the main() function either above or below. Function max has been defined above main() as a separate entity, and has been called from the main() Function max has been defined underneath main() ,and has been called from the main() A function signature has a return type (data-type or void), a name and a formal parameter list int gradepaverage(string name, int numberofsubjects); parameter list int gradepaverage(string name, int numberofsubjects); void myfunction(int x); void donothing(); int main(void) A function may or may not return a value back to the calling function.