COMP 202 Lecture Notes - Lecture 5: Yogurt, Standard Streams, Eggplant
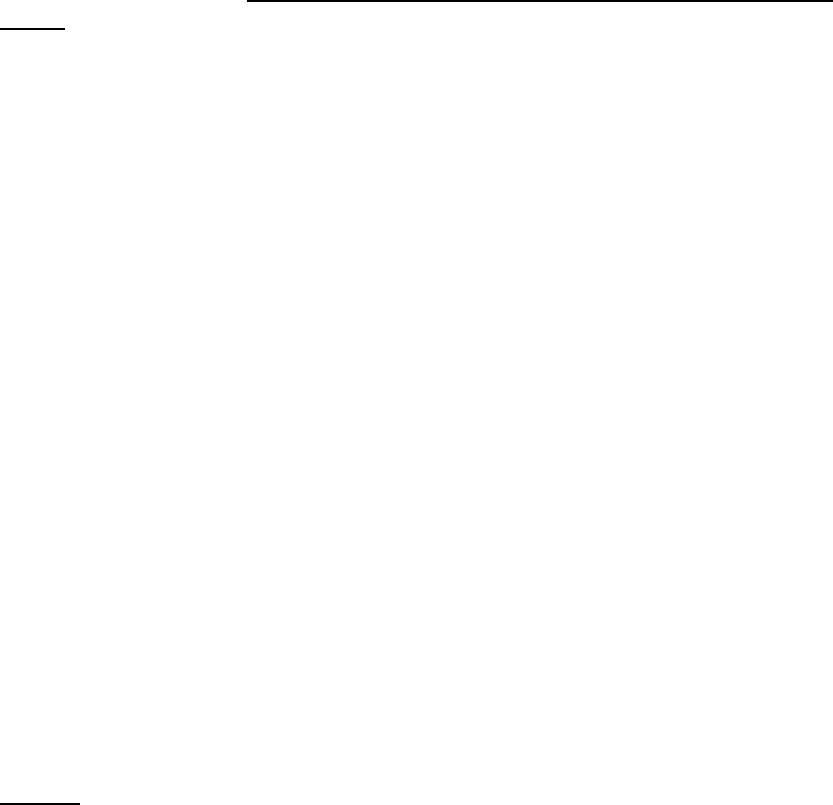
COMP 202: Foundations of Programming
Lecture 5: Arrays, Reference Type, and Methods
Input:
How do we take input from the user?
We need: 1) A place to store it for further computations
2) A way to take input from the user
How do we read input from the user?
At the top of the file write: import java.util.Scanner;
In the main method: Scanner scanner_variable = new Scanner(System.in);
Then, call this method to read a line: scanner_variable.nextLine();
However, we need to store it as a variable.
import java.util.Scanner;
public class Repeater {
public static void main (String[] args){
System.out.println(“Say something”);
//create a scanner to read some input
Scanner scanner = new Scanner(System.in);
String s = scanner.nextLine();
System.out.println(s);
}
}
Now we know how to read a String from standard input – how do we read other variable types?
For the basic data types, use .next<typename>:
scanner.nextBoolean();
scanner.nextByte();
scanner.nextInt();
scanner.nextDouble();
Arrays:
Let’s say we want to ask the user to enter several numbers, and give the mean as output. What should we do?
1) We need to ask the user to enter numbers and store the result into a variable [Scanner]
2) We need to repeat this several times [For Loop]
3) As we go, we should store the sum of the numbers they have entered [Variable]
4) At the end, divide by the total number of numbers they entered [Store # of numbers]
We could store the 100 numbers into 100 different, unrelated variables, but this is time-consuming and error-
prone. As well as this, how would we loop through these 100 different variables?
Arrays: variables that are associated with a list of values instead of a single value
- Are indexed lists, where every element (value in the list) exists at a particular position
- All elements in an array must be of the same type
→ Could also be described as a container object that holds a fixed number of values of a single type
The length of an array is established when the array is created, and after its creation, the array length is fixed.
The first element of an array is at index 0 ([0])
find more resources at oneclass.com
find more resources at oneclass.com
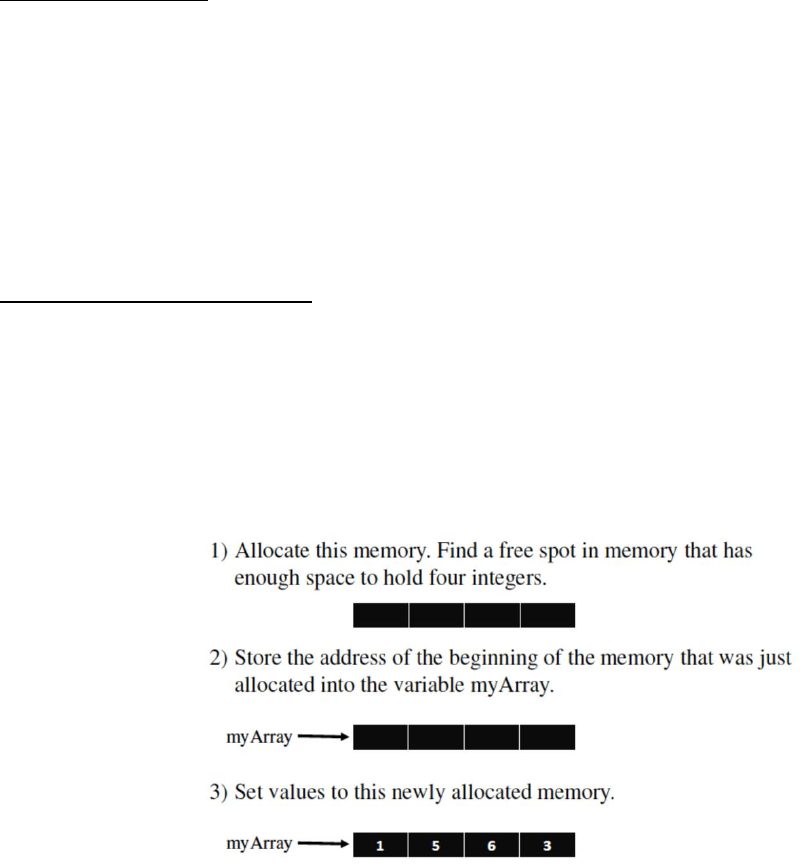
COMP 202: Foundations of Programming
Arrays – Declaration:
Before using an array, you need to declare it as a variable. Here, the square brackets ([]) mean: this variable is
an array whose elements are of a specified type
int[] numbers;
double[] moreNumbers;
String[] namesOfCats;
String[] args;
→ This solves a mystery about the headers we use when writing code – in the line
public static void main (String[] args);
We are declaring a String array called args, that holds program arguments
Putting Information in an Array:
With arrays, you can assign values to all elements when you declare an array
String[] groceries = {“Apples”, “Eggplant”, “Bread”, “Broccoli”,
“Cereal”, “Milk”, “Yoghurt”};
groceries is an array of String values of length 7.
What is the index of Apples? 0
What is the index of Yoghurt? 6
int[] myArray = {1, 5,
6, 3};
Alternatively, you can initialize the array to have a certain size, and then populate the entries one at a time later
on.
Arrays are initialized using the new keyword.
String[] groceries = new String[7];
groceries[1] = “Eggplant”;
groceries[6] = “Yoghurt”;
The length of an array cannot be changed after it has been assigned. Elements in the array can be accessed using
the name of the array and the index of the elements inside square brackets
myArray[3] = 10; //assigns value 10 to myArray at index 3
int x = myString[2]; //assigns value of myArray at index 2 to the variable x
myArray[0] = myArray[3]; //assigns value of myArray at index 3 to myArray at index 0
find more resources at oneclass.com
find more resources at oneclass.com

100
COMP 202 Full Course Notes
Verified Note
100 documents
Document Summary
2) a way to take input from the user. At the top of the file write: import java. util. scanner; In the main method: scanner scanner_variable = new scanner(system. in); Then, call this method to read a line: scanner_variable. nextline(); We need: 1) a place to store it for further computations. However, we need to store it as a variable. Let"s say we want to ask the user to enter several numbers, and give the mean as output. What should we do? public static void main (string[] args){ For the basic data types, use . next: scanner. nextboolean(); scanner. nextbyte(); scanner. nextint(); scanner. nextdouble(); [store # of numbers: at the end, divide by the total number of numbers they entered. We could store the 100 numbers into 100 different, unrelated variables, but this is time-consuming and error- prone. Arrays: variables that are associated with a list of values instead of a single value. Are indexed lists, where every element (value in the list) exists at a particular position.