ENG1003 Lecture Notes - Lecture 5: Mutator Method, Asteroid Family, Javascript
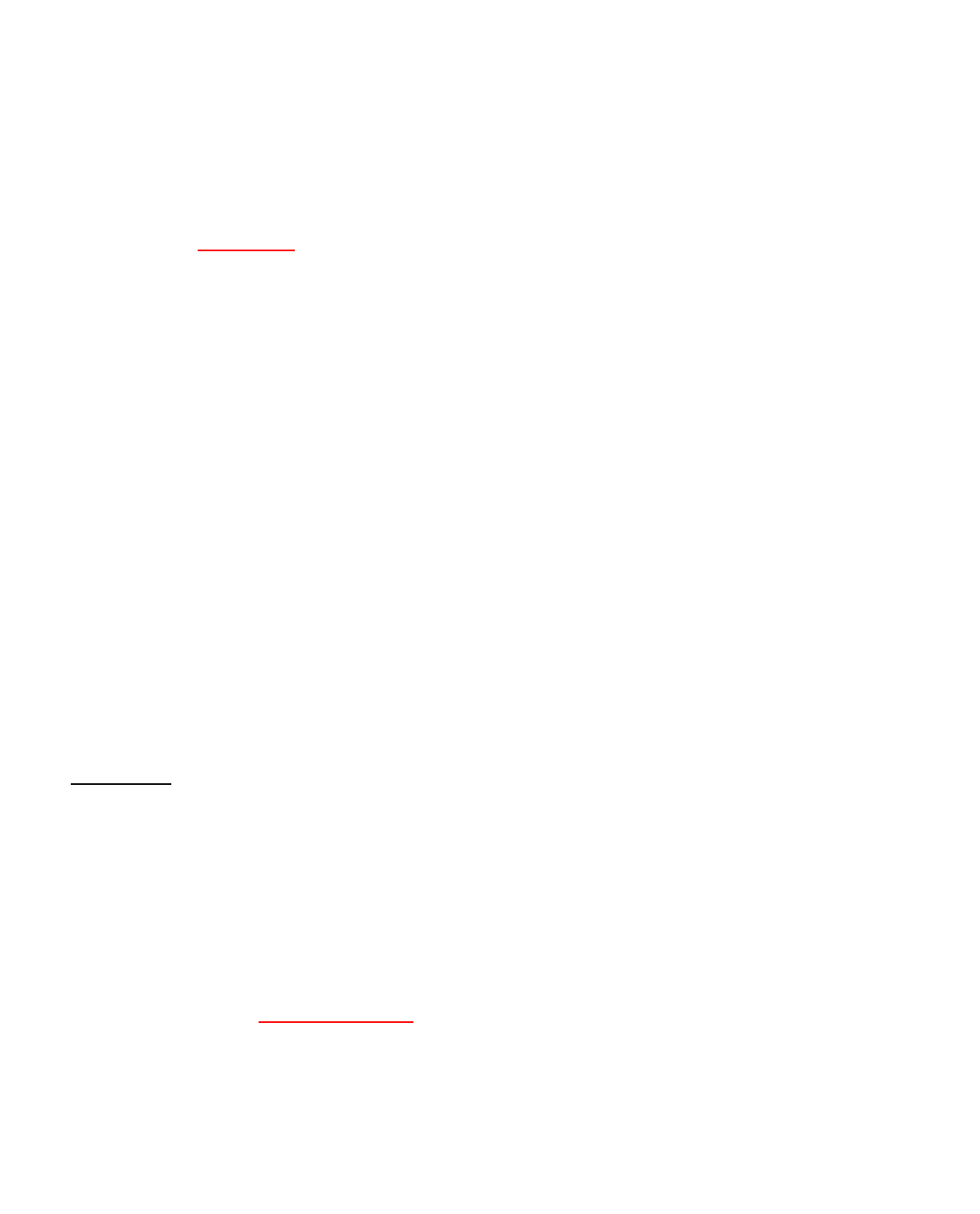
Classes
Ways%to%create%objs%containing%data%
items%(attributes)%&%functns%that%op%
on%this%data%(methods)
•
Encapsulate%related%functns%w/%data%
they%operate%on%-->%protecting%frm%
modificatn%by%outside%world%while%
allowing%use%via%well-def%input%&%
output%pipelines
•
Always%named%with%
UpperCamelCase
•
Instantiation
Process%of%creating%class%instances%=%
objs%that%have%same%set%of%methods%
and%properties%(but%diff%values%for%
those%props)
•
Using%var and new
Eg.%
var
accountA =
new
BankAccount(1000)
○
•
Attributes
Private%instance%variables
To%denote,%name%
attributes/methods%with%_
Eg.%_distance,
this._balance
§
○
•
Priv%attributes%can't%be%changed%by%
someone%outside%code
•
Accessed%via%public%methods of%the%
class,%which%ensures%
access/modificatn%of%the%private%
attributes%in%a%controlled%way
Therefore,%>%common%to%write%
public%methods%that%both%set%
and%get%value%of%instance%
variable
○
•
Eg.%deposit &%withdrawal
public%methods%of%a%bank%account%
class%wld%access%private%balance
attribute%of%indiv%bank%account%objs,%
but%-ve%deposits%can%be%rej%&%there%
can%be%limit%on%withdrawals%within%a%
daily%period%etc.
•
Public%methods (of%a%class)
Constitute%class'%interface (=%a%set%of%
methods%that%can%be%called% by%code%
outside%the%class)
•
Code%outside%class%MUSTuse%this%
interface%to%get%instances%of%class%to%
do%work
•
*Interface%&%implementation of%a%
class%are%separate -->%implem%cld%be%
completely%rewritten,%and%as%long%as%
interface%remains%same,%outside%
world%can%continue%to%use%instances%
of%class%w/o%change
•
Users%of%class%only%need%to%
understand%what%interf%methods%do,%
including%parameters%req%and%values%
returned%-->%instances%of%a%class%can%
be%productively%used%w/o%
unnecessary%overhead%of%
understanding%how%class%internally%
works
•
Benefits
Self-contained%-->%all%code%
designed%to%directly%access%the%
data%of%class%objs/instances,is%
in%the%class
Code%outside%class%shld%
only%call%public%methods%
of%the%class%that%are%
written%in%such%a%way%
that%protects%integrity%of%
the%attributes
○
NOT%access%private%
attributes%of%class%
instances%directly
○
•
Amnt%of%code%that%needs%to%be%
created,%tested,%understood%@%
any%1%time%limited%to%a%class,%
not%whole%system%-->%higher%
productivity%&%lower%error%
rates
•
Lack%of%req%code%context%-->%a%
class%can%easily%be%reused%frm%
one%software%proj%to%
another%-->%saving%time%and%
effort%to%completely%start%frm%
scratch,%allows%them%to%use%
tried%and%tested%classes%frm%
prev%projs
•
Implementation%can%be%easily%
modified%w/o%altering%interf%-->%
requires%no%change%for%code%
that%uses%the%class
•
In-Javascript
Classes%declared%using%class and%constructor keywords
Constructor
Special%method%that%initialises% private%attributes of%class%instance•
May%also%contain%any%no.%of%additional%methods%written%as%functns%within%
class%body,%just%w/o%functn%keyword%usually%used%when%declaring%functns
•
Can%be%used%to%create%a%consistent%object/object%with%a%consistent%set%of%
properties
That%are%then%assigned%attribute(s)%(data%property)%and%method(s)%
(functions)
○
•
When%instantiation%happens,%called%to%initialise% private%attributes•
To%ref%attributes%or%call% methods,%from%code%outside the%class%declaration,%use:
Dot%syntax%w/%variable%containing%a%ref%to%class%instance
Eg. accountA.getBalance()
To%ref%attributes%or%call% methods,%from%code%inside the%body%of%any%method%of%
class,%use:
Dot%syntax%with this keyword%to%refer%to%current%instance
Eg.%this._balance
Getters (accessors) =%public%methods%that%get%value%of%priv%attributes
**get%value%VS%getValue.
Eg.%getBalance()
•
Allows%other%code%to%ref%to%_distance%attribute%using%
accountA.distance instead%of%accountA.distance(),%because%
method%now%has%ability%to%be%used%like%an%obj%property%for%getting%
attribute
•
Methods%marked%as%such%shld%have%no%parameters%and%return%a%single%
value
•
Setters (mutators) =%public%methods%that%set%value%of%priv%attributes,%in%a%
controlled%way
Eg.%setBalance(newBalance)
•
Gives%method%ability%to%be%used%like%an%obj%property for%setting%an%
attribute
•
Mutator%method%can%validate%a%new%value%b4%modifying%a%class'%priv%
attribute,%or%prevent%change%entirely
•
Methods%marked%as%such%shld%have%single%parameter%(new%value%for%the%
attribute)%and%return%nothing
•
Inheritance =%a%way%to%specify%that%a%class%inherits%all%attributes%and%methods of%
a%particular%base%class
Eg.%class%=%Vehicle;%attributes%=%speed,%acceleration,%braking;%
method%=%timeToDestination()
•
Bus,%Motorbike and%Car classes%cld%all%inherit%three%attributes%&%
method,%and%set%their%own%values%for%the%attributes%while%
timeToDestination() remains%unchanged
•
ALSO%EXTENDS.%Eg. class [child class name]
extends
[parent class name]
{
super([call constructor
of parent class eg.
age/11 (as an eg)])
}
•
Polymorphism =%concept%of%code%that%uses%the%same%interf%to%op%on%diff%
types%of%objs%in%a%general%way
Created%by%subclassing%obj%and%overriding%orig%summary method
•
When%summary%method%is%called%for%any%instance,%resulting%in%a%call%to%
the%correct%ver%of%the%method%for%each%instance
Code%of%loop%is%generic,%has%no%idea%of%actual%class%of%each%
instance%in%array
○
•
**
JS%isn't%truly%class-based;%
uses%objs%to%implement%
classes
Functn%can%be%used%
w/%new%keyword%to%
construct%&%return%
new%objs
○
Explains%how%JS%can%
create%class%instances%
&%give%them%methods%
using%functns%stored%
in%obj%properties
○
•
If%no%toString,
[object Object]
-meant%for%when%object%needs%to%
be%a%string.
Encapsulation**,%simpler%to%
work%with%(pieces)
Week$5:$CLASSES$aka$Object$Orientation
Saturday,%25%March%2017
23:00
Document Summary
Ways to create objs containing data items (attributes) & functns that op on this data (methods) Encapsulate related functns w/ data they operate on --> protecting frm modificatn by outside world while allowing use via well-def input & output pipelines. Process of creating class instances = objs that have same set of methods and properties (but diff values for those props) Priv attributes can"t be changed by someone outside code. Accessed via public methods of the class, which ensures access/modificatn of the private attributes in a controlled way. Therefore, > common to write public methods that both set and get value of instance variable. Self-contained --> all code designed to directly access the data of class objs/instances is in the class. Code outside class shld only call public methods of the class that are written in such a way that protects integrity of the attributes. Not access private attributes of class instances directly.