CMPT 166 Lecture Notes - Lecture 17: Graphical User Interface, Boolean Function, Negative Number
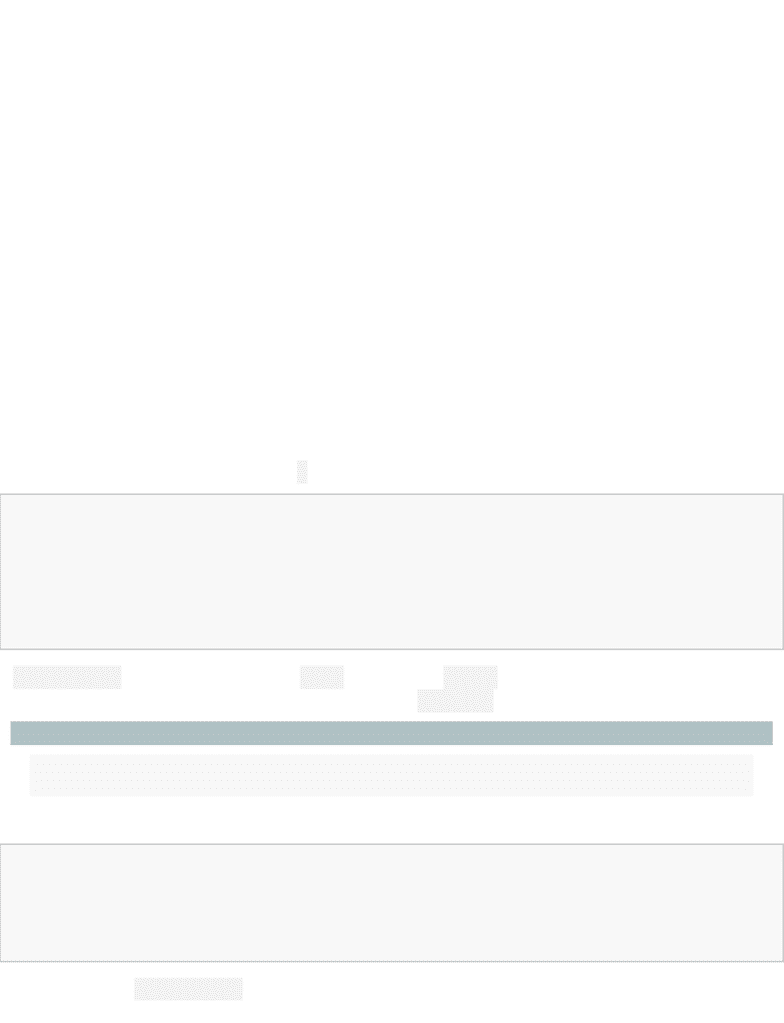
Hit Detection and Functions
In these notes you will learn:
• What boolean functions are.
• How to test if a point is in a circle.
• How to test if a point is in a rectangle.
Introduction
In this note we will see how to do basic hit detection. The general problem is to determine when two
shapes touch, or intersect, each other. For example, in a graphical user interface you need to know
which window the mouse pointer is in when it is clicked. Or in a first-person shooter video game you
want to know when a bullet has hit another player.
Hit detection turns out to be a surprisingly complex topic if you are dealing with arbitrary shapes. Thus,
we will restrict ourselves to a couple of the most important cases involving circles and rectangles.
Boolean Functions
Suppose you want to test if a number, x, is negative. You could do that with this function:
boolean isNegative(float x) {
if (x < 0) {
return true;
} else {
return false;
}
}
isNegative returns either the value true, or the value false. These two values are the boolean
values, and so we call a function that returns booleans boolean a boolean function.
Note
Sometimes boolean functions are called predicates, or test functions. In this course, we will stick with the
term boolean function.
You can use it like this:
if (isNegative(x)) {
println("Can't take the square root of a negative number.");
} else {
println(sqrt(x));
}
The body of the isNegative function consists of an if/else structure. However, some programmers
prefer writing it is this way:
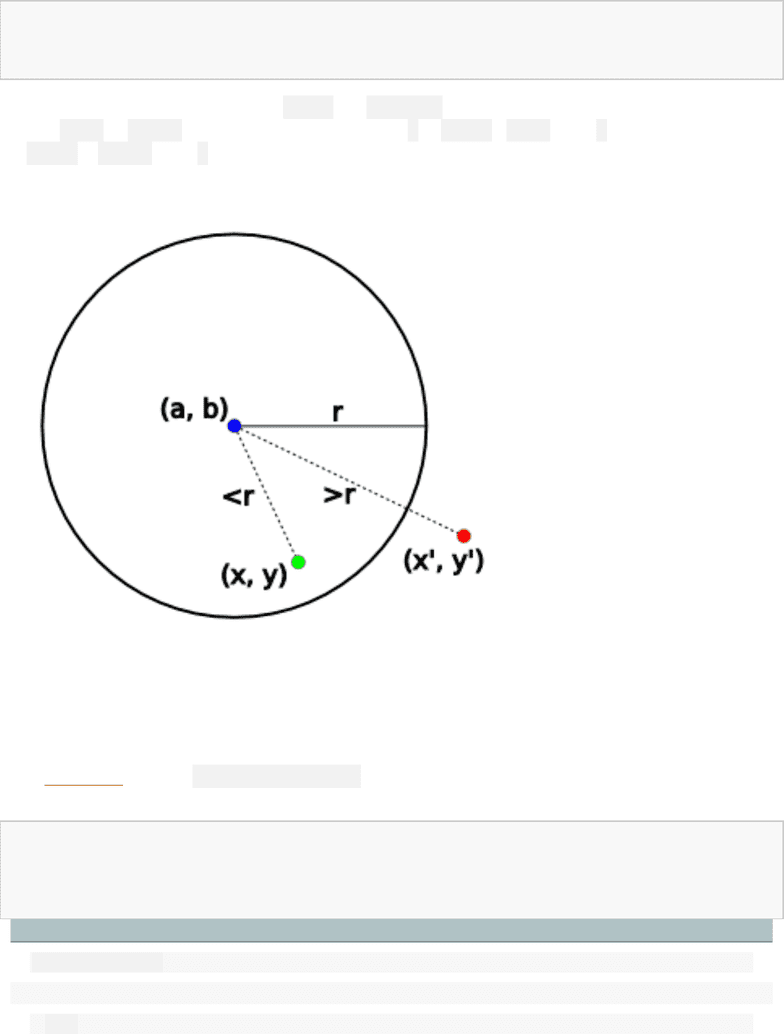
boolean isNegative(float x) {
return x < 0;
}
This is much shorter! The expression x < 0 is a boolean expression that evaluates to
either true or false, depending upon the value of x. If x < 0is true, then x must be negative.
If x < 0 is false, then x is either 0 or positive.
Testing if a Point is in a Circle
Suppose you are given a point (a, b), and a circle with center (x, y) and radius r. How can you tell if (a, b)
is inside the circle, or outside the circle?
As shown in the diagram on the right, the trick is to measure the distance between (x, y) and (a, b). If
it’s r, or less, then (a, b) is in the circle; otherwise it’s outside the circle.
The Processing function dist(x, y, a, b) returns the distance between (x, y) and (a, b). We can
use it like this:
if (dist(x, y, a, b) <= r) {
// (a, b) is in the circle
// do something!
}
Note
dist(x, y, a, b) calculates the distance using this formula:
(x−a)2+(x−b)2‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾‾√(x−a)2+(x−b)2
If dist didn’t already exist, you could could calculate the distance between (x, y) and (a, b) like this:
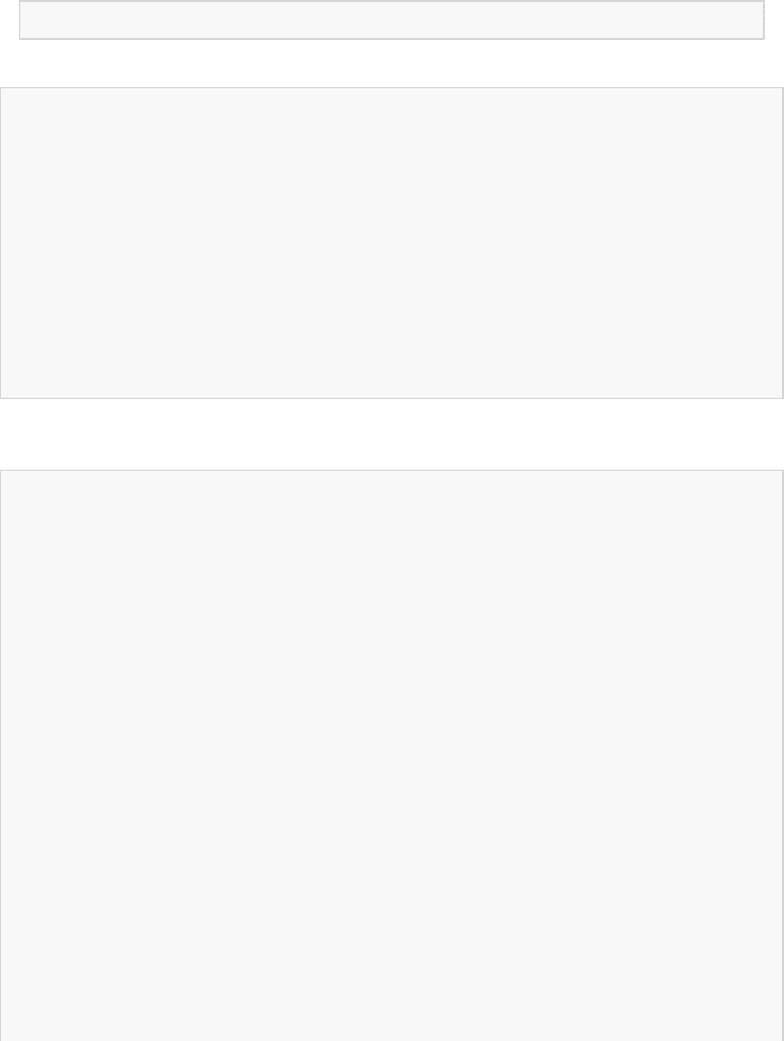
sqrt((x - a) * (x - a) + (x - b) * (x - b))
Let’s write it as a boolean function to make it easy to re-use:
//
// Returns true if (a, b) is in the circle with center (x, y) and with
// radius r; otherwise it returns false
//
boolean pointInCircle(float a, float b, // (a, b) is any point
float x, float y, // (x, y) is the circle's center
float r // r is the circle's radius
)
{
if (dist(a, b, x, y) <= r) {
return true;
} else {
return false;
}
}
For example, here’s a program that changes a bouncing ball’s color to red when the mouse pointer
hovers over it:
class Sprite {
float x;
float y;
float dx;
float dy;
}
//
// Returns true if (a, b) is in the circle with center (x, y) and with
// radius r; otherwise it returns false
//
boolean pointInCircle(float a, float b, // (a, b) is any point
float x, float y, // (x, y) is the circle's center
float r // r is the circle's radius
)
{
if (dist(a, b, x, y) <= r) {
return true;
} else {
return false;
}
}
color ball_color;
Sprite ball = new Sprite();
float diam;
void setup() {
Document Summary
In these notes you will learn: what boolean functions are, how to test if a point is in a circle, how to test if a point is in a rectangle. In this note we will see how to do basic hit detection. The general problem is to determine when two shapes touch, or intersect, each other. For example, in a graphical user interface you need to know which window the mouse pointer is in when it is clicked. Or in a first-person shooter video game you want to know when a bullet has hit another player. Hit detection turns out to be a surprisingly complex topic if you are dealing with arbitrary shapes. Thus, we will restrict ourselves to a couple of the most important cases involving circles and rectangles. Suppose you want to test if a number, x, is negative. You could do that with this function: boolean isnegative(float x) { if (x < 0) { return true;